I am using Nuxt, Vuetify and Storyblok. I have problem when I want to display markdown from storyblok. I try to use Markdownit modul.
First I install it with npm, then import to modules and use it with v-html, but still don't get expected results.
What I am doing wrong?
npm install markdown-it --save
modules: [
['#nuxtjs/markdownit', {
html: true,
linkify: true,
breaks: true,
}],
['storyblok-nuxt', {
accessToken: process.env.NODE_ENV == "production" ? "ygL5rmck1lGa42Vaai7x1Qtt" : 'iyPj3vEKmPladyz3zeqKuwtt',
cacheProvider: 'memory'
}]
],
<p v-html="content"></p>
Result
**Bold text**
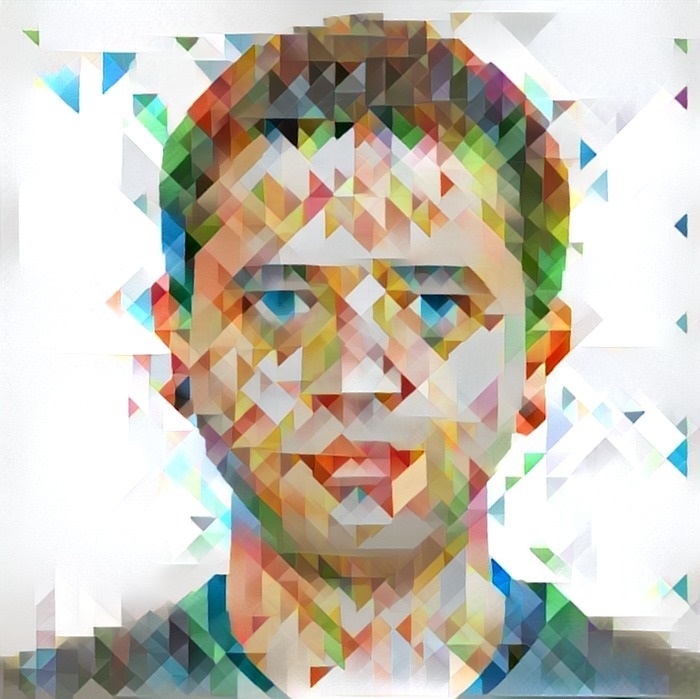
You need to actually use the module, too. You're just importing it at the moment.
What I mean is to write
<p v-html="$md.render(content)"></p>
instead of
<p v-html="content"></p>
Related
I would like to use preinstalled node components in Vuepress (if possible).
Let's say for example I like the following open source framework:
link
And I install it in my Vuepress project
npx create-vuepress-site
cd docs
npm install
npm run dev
npm install #telekom/scale-components#next
Further I add in my config.js the following code in order to use the components:
head: [
['link', { rel: 'stylesheet', href: './node_modules/#telekom/scale-components/dist/scale-components/scale-components.css' }],
['script', { type: 'module', src: './node_modules/#telekom/scale-components/dist/scale-components/scale-components.esm.js' }]
],
I would now assume that I could simply use the components, e.g.
<div>
<scale-button>Click!</scale-button>
</div>
Unfortunately through, it just returns plain text.
Is there a step in between that I am missing?
I have recently switched to laravel-mix from Vue-CLI.
All my images in html stopped working. They were used like this:
<img src="#assets/images/logo.png" alt="Logo">
(#assets is a alias for the resources/assets folder)
With this method, when I look at the html in the browser, I see that it got compiled to [object Module]. So I was able to fix it this way:
<img :src="require('#assets/img/sidebar/logo.png').default" alt="Logo">
But I can't imagine this being the best solution, it seems very hacky and I dont want to add this require().default thing everytime I use an image.
I've tried adding this to the mix.webpackConfig:
module: {
rules: [
{
test: /\.(png|jpe?g|gif)$/i,
use: [
{
loader: 'file-loader',
options: {
esModule: false,
},
},
],
},
]
}
But that doens't do anything, but I don't even know if it works at all to be honest.
Is there a better way to use images with laravel-mix or is require().default really the way to go?
Laravel-mix offers the ability to configure aliases
const path = require('path');
mix.alias({
'#assets': path.join(__dirname, 'resources/assets')
});
I have purchased this VUE template. From its documentation, if I run npm run install && npm run dev. It looks correct
When I execute npm run build to get my production ready project. As you can see, it does not look the same:
I have checked the css links inside of the generated HTML, and there is only one pointing to "a file". I believe is the right one...(?)
Here is vue.config.js:
module.exports = {
publicPath: process.env.NODE_ENV === 'production' ? './' : './',
outputDir: 'dist',
assetsDir: 'app',
indexPath: 'index.html',
configureWebpack: {
devtool: 'source-map'
},
}
My env.production looks like:
NODE_ENV=production
VUE_APP_TITLE=My Base Front End
What am I missing on the config file?
Try adding below code for tag stylesheet_pack_tag in your layout file :
<%= stylesheet_pack_tag 'application', media: 'all', 'data-turbolinks-track': 'reload' %>
I can recall similar issue from past experience.
Vue component
<ckeditor :editor="classiceditor" v-model="report_notes" :config="editorConfig" #blur="editReportNotes()" #keyup.enter="editReportNotes()"></ckeditor>
vuejs code
classiceditor:ClassicEditor,
editorConfig: {
// plugins: [ Underline],
toolbar: {
items: [
'bold','italic',
'|','link',
'|','bulletedList', 'numberedList',
]
},
placeholder :'Write a note...',
link: {
defaultProtocol: 'http://'
}
},
Issue is defaultProtocal is not setting. when i give gogole.com as link, It is opening as my-domain/google.com which is a non existing page
I need it as http://google.com
I followed this doc defaultProtocal
Thank you in advance
re-installeed and Upgraded from version 16.0.0 to 22.0.0. Because the feature is recently added
npm install --save #ckeditor/ckeditor5-vue #ckeditor/ckeditor5-build-classic
How can I properly setup linting with prettier and the vue linters with running into conflicts in vue files with and typescript code?
An example:
Default: closing bracket (in this case --> closing li) is in next line.
<template>
<div>
<li
class="o-playtime__head__info__tags__item"
v-if="playtimeItemObject.event.ageRecommendation"
>
...
</div>
</div>
</template>
I want the closing bracket in the same line:
<template>
<div>
<li
class="o-playtime__head__info__tags__item"
v-if="playtimeItemObject.event.ageRecommendation">
...
</div>
</div>
</template>
Eslint configuration:
module.exports = {
root: true,
env: {
node: true
},
extends: [
'plugin:#typescript-eslint/recommended',
'prettier/#typescript-eslint',
'plugin:prettier/recommended',
"plugin:vue/essential",
"#vue/prettier",
"#vue/typescript",
],
parserOptions: {
parser: "#typescript-eslint/parser"
},
};
Prettier configuration:
module.exports = {
semi: true,
trailingComma: 'all',
tabWidth: 2,
};
Adding the vue linting rule to change the closing bracket:
rules: {
"vue/html-closing-bracket-newline": ["error", {
"singleline": "never",
"multiline": "never"
}]
}
This causes a conflict between vue/html-closing-bracket-newline and prettier/prettier.
Is there a way to overrule prettier for this case? Or do I have to configure both vue and prettier?
Or is there a general better way for combining the linters in vue development? I do not like the combination between two different formatters at all.
Is there a way to let different linters handle the different segments of a vue file?
<template> --> Linted by vue/recommended
<script lang="ts"> --> Linted by prettier/recommended // prettier/#typescript-eslint
After digging deeper into all the mentioned linter configurations:
Currently, there is no proper way to combine prettier with vue linting rules without running into conflicts, as prettier does not allow such configurations at the time of writing. It works out of the box, but as soon as there will be other rules defined for vue template handling, both formatters run into conflicts (vue formats after the defined rules, prettier tries to overwrite it again).
In my opinion, prettier does a good job, but is not a good partner to run beside other formatters due to missing configuration / skipping options. So in my case I removed prettier as a formatter and restrict linting to pure eslint with eslint-typescript and eslint-vue rules. This is a bit more work in configuration, but allows more customized formatting / linting without any conflicts.
eslint conf:
extends: [
"plugin:vue/recommended",
"eslint:recommended",
"#vue/typescript/recommended"
],